這一篇主要是要記錄一下反射(Reflection)的方便用法,作為以後的備忘。底下的範例會順便用一些Depency Injection的觀念來實作。
根據MSDN的描述#
反映 (Reflection) 會提供 Type 型別的物件,用來描述組件、模組和型別。 您可以使用反映來動態建立型別的執行個體、將型別繫結至現有物件,或從現有物件取得型別,並叫用其方法或存取其欄位和屬性。 如果您在程式碼中使用屬性,反映可讓您存取這些屬性。
在動物園裡面有很多不同的動物園區,每個動物園區都可以看到專屬於該園區的動物。當遊客買票進去時,可以依據票的種類去不同的園區觀賞。假設目前我們有兩個園區:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
| /// 獅子王動物園區
public class LionKing
{
private ICollection<string> _Pool;
public LionKink()
{
_Pool = new List<string>{
"Simba",
"Nala",
"Pumbaa",
"丁滿"
};
}
public void SeeAnimals()
{
foreach(var name in _pool)
Console.WriteLine("在動物園區我看到 {0}."name);
}
}
/// 憤怒鳥動物園區
public class AngryBird
{
private ICollection<string> _Pool;
public AngryBird()
{
_Pool = new List<string> {
"RedBird",
"BlueBird",
"YellowBird"
};
}
public void SeeAnimals()
{
foreach(var name in _pool)
Console.WriteLine("在動物園區我看到{0}."name);
}
}
|
方法: 通常看到這樣的需求有一種寫法是最簡單直覺的:
1
2
3
4
5
6
7
8
9
| class Program
{
static void Main(string[] args)
{
AngryBird ab = new AngryBird();
ab.SeeAnimals();
Console.Read();
}
}
|
但你我都知道這樣以後動物園區一多,不但要新增該新增的類別,連呼叫端都要一起改,這樣有點不太方便。有鑑於每個園區提供的服務都差不多,都是讓遊客觀賞的,所以我們需要一個可以把這個服務或是說功能提煉出來的類別,讓這些園區都繼承這個類別,而遊客只需依賴這個類別就好,至於實際要去看哪個園區(或是說實際使用的是哪一個服務)則交由子類別本來決定。依據上述的需求我們可以把SeeAnimals() 這個方法提煉出來,於是有了底下的類別:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
| /// <summary>
/// 動物園區。
/// </summary>
public abstract class AnimalZone
{
/// <summary>
/// 動物園區的名稱。
/// </summary>
public string ZoneName { get; set; }
/// <summary>
/// 展示動物園區的所有動物。
/// </summary>
/// <returns></returns>
public abstract ICollection<string> ShowZoneAnimals();
}
|
(ps.我知道這個時候這邊變成吐出一個泛型集合有點怪,但是請繼續看下去吧~)
而我們的兩個園區就可以改寫成這樣:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
| public class LionKing:AnimalZone
{
private ICollection<string> _Pool;
public LionKing()
{
this.ZoneName = "獅子王園區";
_Pool = new List<string> {
"Simba",
"Nala",
"Pombaa",
"丁滿",
"Scar",
"Monky"
};
}
public override ICollection<string> ShowZoneAnimals()
{
return _Pool;
}
}
public class AngryBird:AnimalZone
{
private ICollection<String> _Pool;
public AngryBird()
{
this.ZoneName = "憤怒鳥園區";
_Pool = new List<String>{
"RedBird",
"BlueBird",
"YellowBird",
"BlackBird",
"GreedBird",
"WhiteBird"
};
}
public override ICollection<string> ShowZoneAnimals()
{
return _Pool;
}
}
|
好~但是還不夠,讓他們繼承個抽象類別事情還沒有結束,改到目前為止做到一半了。剩下的就是用另外一個類別把這個變化封裝起來,我們讓這個類別依賴 AnimalZone 這個抽象類別,然後由這個類別去執行 AnimalZone 提供的服務,這樣我們以後只需要知道這個類別就可了,或是說服務更動時就去改這裡。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
| public class AnimalFactory
{
private AnimalZone _animalZone;
/// <summary>
/// 建構子,依據傳入的值決定要去哪個園區。
/// </summary>
/// <param name="animalzone">動物園區名稱。</param>
public AnimalFactory(string theAnimalzone)
{
if(theAnimalzone=="LionKing")
this._animalZone = new LionKing();
else
this._animalZone = new AngryBird();
}
/// <summary>
/// 看動物去。
/// </summary>
public void SeeAnimals()
{
foreach (var animal in this._animalZone.ShowZoneAnimals())
{
Console.WriteLine("I see {0} in {1} zone.", animal, _animalZone.ZoneName);
}
}
}
|
之後在用戶端就可以依據傳入的字串來決定可以看到哪些動物,其實若動物園經費不足以後不會擴大(咦?!)的話這樣寫就算結束了。但是事情很多時候不像我們想的這麼美好,若以後動物園園區越來越多,建構子中的判斷式或是 switch case 就會讓程式變得難以維護,若可以把這討厭的判斷消滅該有多好….。這種時候就是 Reflection(反射) 登場當英雄的時候啦!
我們可以藉由反射達成動態的產生實體,不需要 if else 或是 switch case 惱人的判斷了!實際上的改寫方法如下:
這邊我們只需要修改建構子的部份
1
2
3
4
5
6
| public AnimalFactory(string theAnimalzone)
{
var myType = Type.GetType(theAnimalzone);
var animalzone = Activator.CreateInstance(myType) as AnimalZone;
this._animalZone = animalzone;
}
|
我們就只需要這樣短短三行,就可以把未來一卡車的判斷給消滅了!這邊要講一下傳入的字串會像是這樣:YourNameSpace.LionKing
這邊用到主要的類別是 Activator ,用這個可以建立物件型別,而他的 CreateInstance 方法除了可以接受無參數的建構式之外,還支援附帶參數的建構子。藉由這種方式我們在呼叫端只需要輸入相對的型別名稱的字串,就可以直接生出相對應的類別了。呼叫端也只需要知道AnimalFactory 這個類別,而 AnimalFactory 也只依賴 AnimalZone 這個抽象類別。
整個類別的架構圖會長這個樣子:
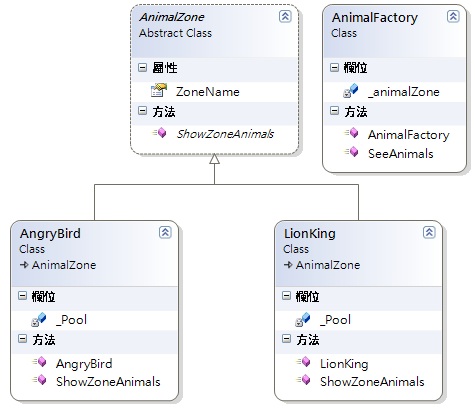
另外要提醒的是….AnimalFactory 的建構子應該要捕捉例外的…只是我這次偷懶偷很大沒有做,若真的要實際應用的話這是一定要做的。
這算是一個簡單的設計模式的練習,搭配反射讓應用更為有彈性。這邊我們也可以發現到把變化封裝起來是一個在設計系統時必須考慮到的點,只要可以控制住變化,系統的維護負擔就會相對變小,以後有機會的話會多以設計模式搭配來寫文章。
參考文獻#